Mastering React State Management: Tips & Tricks
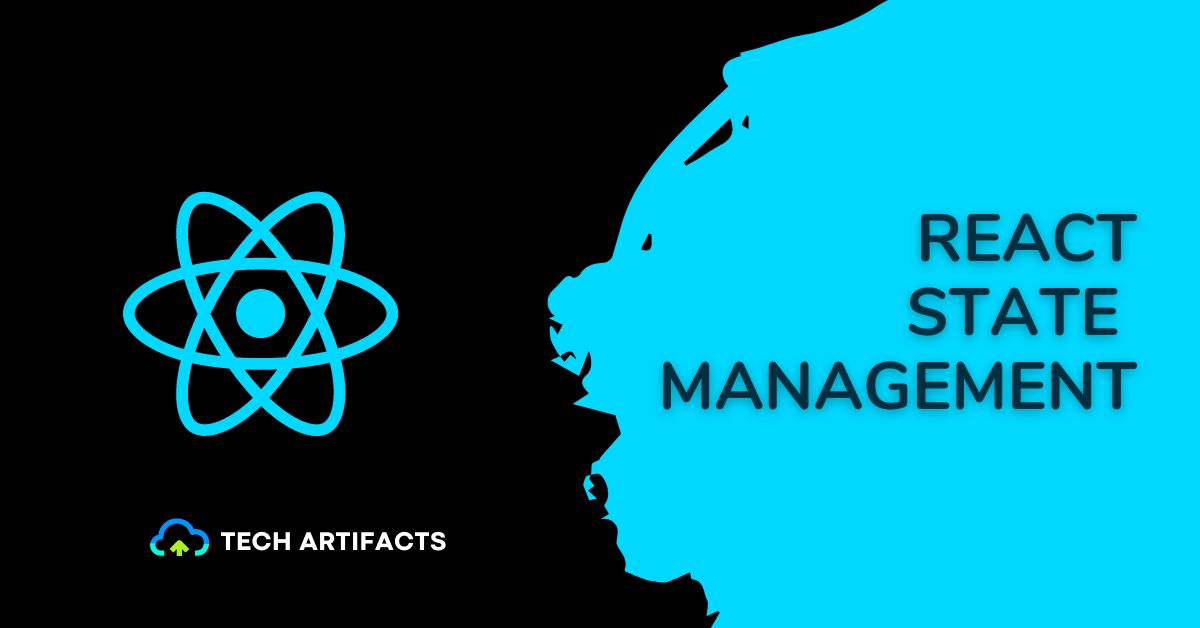
Hello, Welcome to Tech Artifacts. I hope you are doing well.
In this blog, we are going to cover the topic of React State Management. State management is the process of maintaining and updating the data or state of a client-side application over time.
If you are new to React, I would recommend you first take a look at the below blogs โ
State Management
Letโs first understand what is state management.
In client-side applications, state refers to the data that is used by the application to render its user interface (UI). This data can include information such as user input, API responses, and other data that changes dynamically over time.
State management is an important aspect of building client-side applications. It ensures that the UI remains up-to-date and consistent with the change in the underlying data.
Without proper state management, it can be challenging to keep track of the data used by an application. The state also helps to ensure that the UI remains in sync with this data.
Importance of Statement Management in React
Improves Consistency
In React, State Management enables developers to manage the data used to render the user interface. It also helps to keep track of the changes made to this data over time. This helps to prevent inconsistencies in the UI and to ensure that the application remains in a consistent state.
Improves Maintenance and Scalability
State management also makes it easier to maintain and scale applications. It provides a centralized approach to managing the state of the application. This allows developers to manage the state of the application in a more organized and predictable manner. To make changes to the state without affecting other parts of the application.
Improves Application Performance
State management can also help to improve performance by reducing the amount of data that needs to be re-rendered when changes are made to the state. By only updating the components that are affected by the changes to the state, React can optimize the performance of the application and provide a more efficient user experience.
Example of Statement Management in React
The importance of state management in React can be best explained through an example.
Consider a simple React application that displays a list of to-do items. Each item in the list has a Title, a Description, and a Status that can be either โcompleteโ or โincomplete.โ
Without state management, you would have to manage the state of each to-do item individually in the component that renders it. This would quickly become complicated and difficult to maintain as the number of to-do items grows.
With state management, however, you can manage the state of all to-do items in a single place. We can create a State Object in the parent component. The state object contains the data for each to-do item and is updated when changes are made to the to-do items.
This centralized approach also makes it much easier to maintain the application, as you only need to update the state object in one place, and the changes will be automatically reflected in the UI. For example, if a to-do itemโs status changes from โincompleteโ to โcomplete,โ you only need to update the state object to reflect this change, and the UI will be automatically updated to show the new status.
โuseStateโ Hook
useState
is a hook in React that allows you to add a state to your functional components.
Itโs very easy to use the hook useState
. See the below code block โ
import React, { useState } from "react";
const ExampleComponent = () => {
// Declare a state variable named "count" and initialize it to 0
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
};
export default ExampleComponent;
In this example, we used useState
to create a state variable called count and initialize it to 0. The useState
hook returns an array with two elements: the current value of the state, which is count, and a function to update the state, which is setCount
.
We use the setCount
function to update the count state variable whenever the button is clicked. The count state variable is displayed in the componentโs render output, and it will update whenever the state changes.
useState
is a very important concept in React, and itโs a good idea to become comfortable with it before moving on to more advanced state management techniques.
Other State Management Techniques
As your React application grows and becomes more complex, the useState
hook can become complicated for several reasons:
- Increased complexity of data flows: As your application grows, you may need to manage more complex data flows and relationships between different parts of your state. For example, you may need to manage data dependencies, perform asynchronous actions, or manage multiple pieces of state that are related to each other.
- Managing state across multiple components: When using the
useState
hook, each component has its own state, which can make it challenging to manage state across multiple components. For example, if you need to share the state between components, you may need to pass data through props, which can become cumbersome as your component tree grows larger. - Difficulty with debugging: As your application grows, it can become more difficult to debug state issues. For example, if a componentโs state is not updating correctly, it can be challenging to determine why and fix the issue.
- Maintaining consistency: As your application grows, it can be difficult to maintain consistency in your state management. For example, you may need to manage multiple pieces of state that are related to each other, or you may need to enforce constraints on the state, such as ensuring that certain values are always within a certain range.
To address these challenges, many React developers choose to use a state management library such as Redux or MobX. These libraries provide a centralized approach to managing the state, which can simplify state management as your application grows and becomes more complex.
We will cover more such State Management libraries in my upcoming blogs.
Conclusion
In this blog, we learned how state management is an essential part of building React applications. We looked at how it allows you to store and manage data that can change over time and affect the way your components behave and render information to the user.
We looked at the useState
hook which is a built-in tool that provides a simple way to add state to functional components, and itโs a great starting point for learning about state management in React.
In this blog, we also looked at some other ways of managing states using Redux and MobX libraries which can help manage states on a larger scale. Regardless of which method you choose, understanding state management is critical to building successful React applications.
Thank you for taking the time to read this blog post. We hope that you found the information provided in the blog to be helpful and informative. For similar content, please check out our other blogs.
We appreciate your support and feedback, and we would love to hear from you if you have any questions or comments about the blog. If you have any specific topic you want us to cover in the future, please feel free to let us know.